01/10/2019
Making HTTP requests is one of the most fundamental tasks that you can perform as a web developer. As such, it's important to know how to do them properly in the language of your choice. Having recently made the jump to PHP, it took me a while to understand how these were done. However, now that I've familiarized myself with it, I thought I'd share other newcomers π€ how to do HTTP requests in PHP with cURL, a built-in library.

- The CURL extension to PHP is designed to allow you to use a variety of web resources from within your PHP script. By means of utilizing cURL we are able to send HTTP request utilizing quite a lot of method like GET, POST and we can also be accessing to third party API systems. How to Use PHP CURL?
- Once you've compiled PHP with cURL support, you can begin using the cURL functions. The basic idea behind the cURL functions is that you initialize a cURL session using the curlinit, then you can set all your options for the transfer via the curlsetopt, then you can execute the session with the curlexec and then you finish off your session using the curlclose.
- Most programming languages support cURL commands using some libraries or extension. CURL is one of the popular methods to interact with REST API data. PHP use cURL library (module) to provide access curl functions within PHP. By default, most PHP installations come with cURL support is enabled.
What is cURL?
If you've spent some time in the web development sphere, you might have already heard of it. cURL, which stands for client URL, is an open-source command line tool that is used to transfer and receive data using internet protocols such as HTTP, HTTPS and FTP. Created by Daniel Stenberg in 1997, cURL is used in countless devices ranging from cars π and television sets to tablets and audio equipment. π
So where does PHP come into play? Well, PHP has a module called cURL PHP that allows us to access the functionality offered by this awesome tool. Let me show you next how to use it, 'kay?
1) Open the network tab in DevTools 2) Ctrl-click a request, 'Copy as cURL'. 3) Paste it in the curl command box.
The Code
In this tutorial, I'll be showing you how to do the 2 most common HTTP request methods: GET
and POST
. However, regardless of which method you opt for (even PUT
and DELETE
) , they will all follow the same steps.
- Initialize a cURL session
- Set the URL and other various options for the session
- Execute the request and save the response
- Close the session to free up resources
Seems pretty straightforward, right? π So how would this look like with code?
Redaction software download for mac. Perhaps with the exception of the options setting (which we'll get into next π), everything appears to be quite straightforward. At the end of these 4 steps, you're left with a variable $response
that contains the response of our GET request. This may be JSON, a string or a complete HTML page.
Understanding options
Next to error handling, options are where you will be paying attention most of the time. Options are set with the curl_setopt(resource $ch, int $option, mixed $value)
function, which takes three parameters: the cURL resource/session, the option and its corresponding value.
So you may be wondering: 'So what kind of options can I set?' π€ Ahem.. maannnnyyyy! Too many to even go through one by one. Fortunately, you will only need a handful for most of your requests:
Curl To Php Converter
CURLOPT_RETURNTRANSFER
- Return the response as a string instead of outputting it to the screenCURLOPT_CONNECTTIMEOUT
- The number of seconds to wait while trying to connectCURLOPT_TIMEOUT
- The number of seconds to allow cURL functions to executeCURLOPT_URL
- The URL to fetchCURLOPT_CUSTOMREQUEST
- Set custom request method such asPUT
orDELETE
. cURL defaults toGET
CURLOPT_POSTFIELDS
- An array of the data to send in a requestCURLOPT_HTTPHEADER
- An array of HTTP header fields to set
A handy trick
Now, it doesn't take a genius π€ to realize that you'll be repeating curl_setopt()
quite a bit if you need to set several options. Of course, the creators were well aware of this and have provided us two ways to cut down on the repetitiveness.
First, instead of setting the URL with curl_setopt($ch, CURLOPT_URL, 'dilshankelsen.com')
, we can pass in the URL immediately when initializing the cURL session, like so: $ch = curl_init('dilshankelsen.com')
.
Second, we can use curl_setopt_array(resource $ch, array $options)
to set multiple options at once. An example would look as follows.
Receiving the response
As you surely noticed before, curl_exec($ch)
is responsible for executing your HTTP request. In addition to that, it will return three different responses depending on the following conditions:

false
if the request fails to executetrue
if the request executes without error andCURLOPT_RETURNTRANSFER
is set tofalse
- The result (e.g JSON) if the request executes without error and
CURLOPT_RETURNTRANSFER
is set totrue
Another useful function at your disposable is curl_getinfo($ch)
, which returns information about your request, such as the response code or total transaction time.
Error handling
Errors β happen.. there's nothing you can do about it. But at the very least, you should know how to properly handle them with cURL. For this task, we have two functions: curl_error($ch)
and curl_errno($ch)
. If applicable, the former returns the error message, while the latter returns the cURL error code. The most common way to use these two functions is with an if
statement.
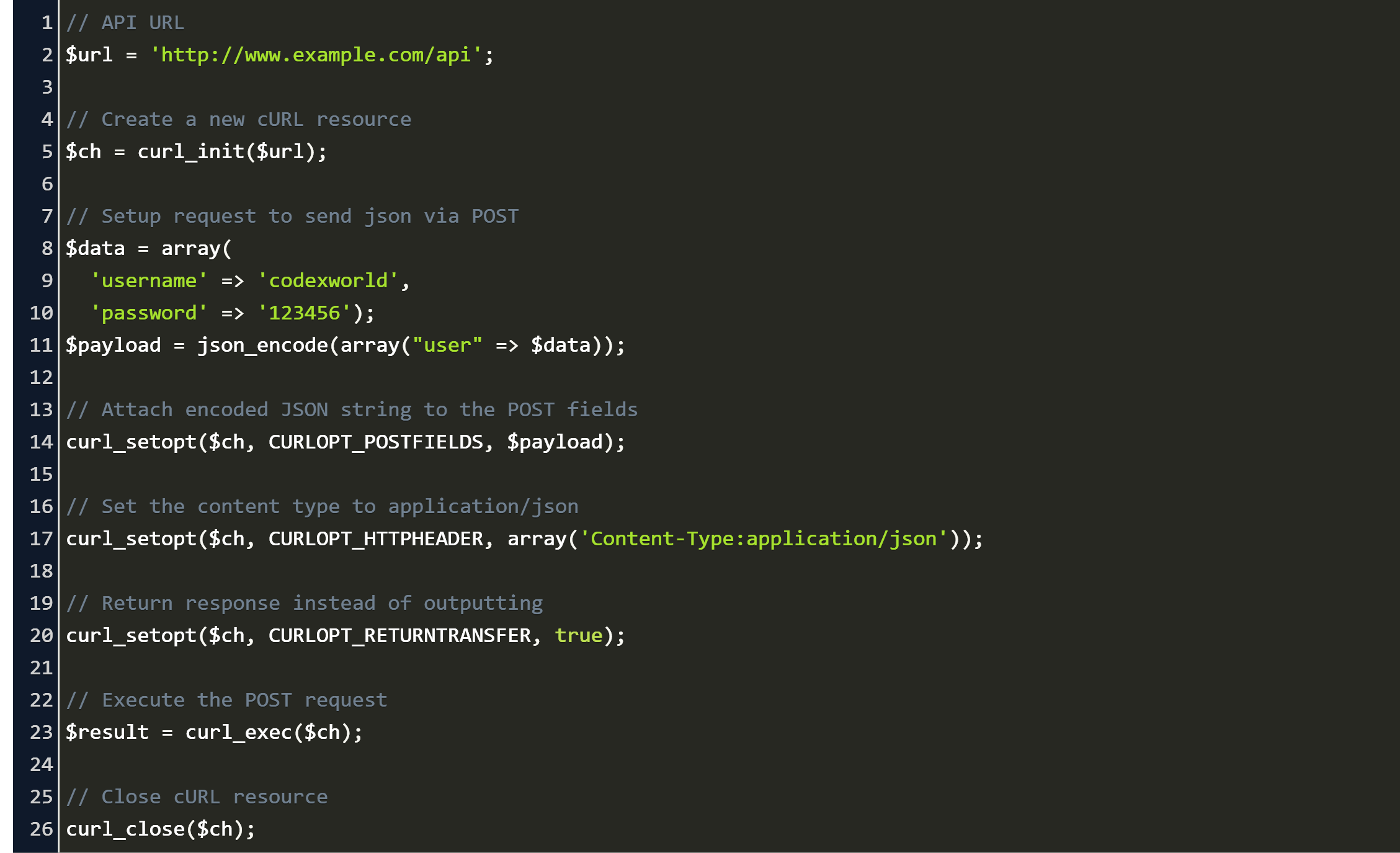
POST request
So far, all examples I've been showing you were GET
requests. In fact, when no request method is specified, cURL simply defaults to GET
. To tell cURL you would like execute a POST
method, simply set the CURLOPT_POST
Download epic medical software for mac. option to true. However, I prefer to use the option CURLOPT_CUSTOMREQUEST
as any method may be specified with it. One less option to remember! π
Without further ado, let's see how you would preform a proper POST
Where-to-download-old-mac-os-software-from. request, with error and JSON handling.
In this example, we attached a payload to our request. For this, we needed to encode our payload and set the appropriate headers in the options. If you're not familiar with JSON handling in PHP, why not check out my article on this topic (shameless plug π ).
The PHP cURL is a library used for making HTTP requests. In order to use PHP cURL, you must have installed and enabled libcurl module for PHP on your system. In this tutorial, you will learn how to POST JSON data with PHP cURL requests. Basically, there are 4 steps involved to complete a cURL request using PHP.
- curl_init β The first step is to initializes a new session of cURL and return a cURL handle to other functions.
- curl_setopt β The second step is to set options for a cURL session handle. All these settings are very well explained at curl_setopt().
- curl_exec β In third step it perform a cURL session based on above options set.
- curl_close β The last step is to close a cURL session initialize by curl_init() and free all resources. Also deleted the cURL handle.
Letβs use the below sample code to create a POST request with PHP cURL.
2 4 6 8 10 12 14 16 18 20 22 24 26 28 30 | // A sample PHP Script to POST data using cURL 'username'=>'tecadmin', ); $payload=json_encode($data); // Prepare new cURL resource $ch=curl_init('https://api.example.com/api/1.0/user/login'); curl_setopt($ch,CURLINFO_HEADER_OUT,true); curl_setopt($ch,CURLOPT_POSTFIELDS,$payload); // Set HTTP Header for POST request 'Content-Type: application/json', ); // Submit the POST request curl_close($ch); ?> |
Php Curl Print Raw Request
The main thing is that the request must be a POST request with properly json-encoded data in the body. The headers must properly describe the post body.
